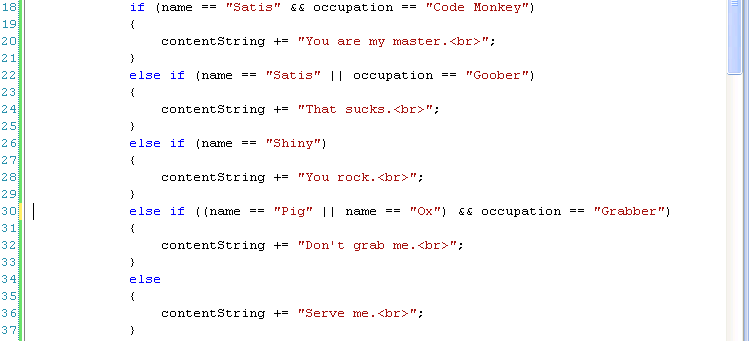
If-Then-Else More Complicated
There's a lot more to this. Let's go over it peice by peice. First, you may notice it's not just if - then - else. In this case it's if - then - else if - else if - else if - else. The else if statements basically let you test a whole range of different statements. The first thing we do is check if name == "Satis" && occupation == "Code Monkey". The && means "and". Basically if the name is set to Satis and the occupation is set to Code Money, we append the "You are my master.<br>" string to contentString and then continue executing code. Note, we do NOT keep checking through the else ifs. Once you match one, you're done.
If the name is "Satis" and occupation is not "Code Monkey", we check the next statement. Now we're seeing if the name is "Satis" (again). However, intead of && we have ||. || means or. Thus if the name is Satis or the occupation is Goober, you get "That sucks.<br>" appended to the contentString.
If we still haven't made a match, we check if name == "Shiny" and, if so, we append "You rock.<br>" to the contentString. Pretty straight forward, right?
The next statement has some parenthesis in it. You may remember from math the concept of grouping operations. Basically any operations inside parenthesis are executed first, then operations are executed in order. In this case we're seeing if the name is either "Pig" or "Ox". Once we've checked that, we see if their occupation == "Grabber". If the name is either Pig or Ox and they're Grabbers, we append the "Don't grab me.<br>" string to contentString. What would have happened if we didn't have those parenthesis in there? Due to order of operations the statement would have returned true if the name was Pig, period. The occupation would never have been checked. Meanwhile, Ox would have had to be a Grabber before this statement evaluated as true. When doing statements like this, always remember to group properly. Grouping items that don't necessarily need to be grouped won't cause any problems, but forgetting to group things can cause confusing behavior from your application.
The only time you really need to worry about grouping is when using an or (||) operator. If all you have is and (&&) operators, grouping doesn't really affect much. However, if you're using math in your comparison than group may very well be important. For instance, you could do something like: if((x^2 + 5)/10 >= 8). In this case, we take the variable x, square it, add 5, then divide all of that by 10. If the result is greater than or equal to 8, the if statement is satisfied. However, what happens if you write it without the parenthesis? if(x^2 + 5 / 10 >=8). Since division comes before addition in order of operations, you would get x squared + 2. Big difference.
Finally, if none of the other criteria are met, we return the default response of "Serve me.<br>". Do we need this else on there? No. If you have a series of conditions like above but don't want an else statement, just leave it off. The following is perfectly valid code, though you may notice the last statement lacks parenthesis around the or section. As such, Pig will always match it, while Ox will only match if he's a grabber..
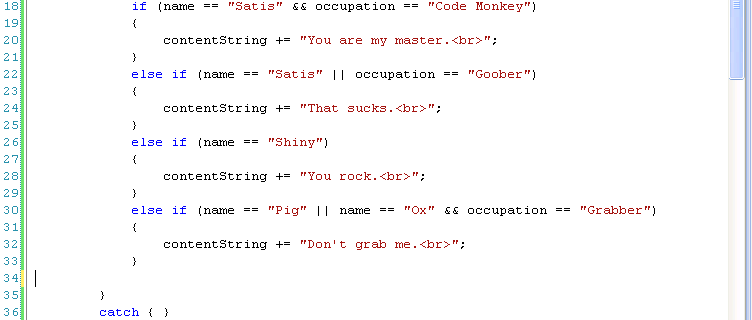
Else Isn't Required
Once again, this is a really important section to understand. If there's any confusion, play around with it. Try matching occupations, names, dates, whatever. On the next page we'll continue with ternary operations, a special version of the if - then - else statement.